Use the Consolidation Server Introspection
Before you begin: Launch a full scan to fill the object graph with data.
-
From Consolidation Server, select the Consolidation
Server instance for which you want to build an object graph.
-
In URI(s), select node URIs to generate the object
graph starting from these nodes. It can be helpful to filter on the node
type.
-
In Max. depth, specify the maximum exploration depth of the
graph. The nodes which are beyond this maximum depth are not displayed in the
graph.
-
In Max. arcs per node, specify the width of the object graph.
It takes the n first arcs of each node.
-
From Color, you can switch the highlight of either
Nodes or Arcs.
-
Click Submit to generate and display the object
graph.
-
With the generated object graph, you can:
- Zoom in and out using the + and -
sign or using your mouse wheel, and also pan the view.
- Click Export to export the graph to a DOT file. You can
also do that with the
cvdebug command line tool. See Exporting the Object Graph.
- Double-click a node to define it as the new root of the object graph.
- Click a node to view its details. In the Node details
panel, you can then:
Possible Action
|
to ...
|
Click Force aggregation
|
Start the aggregation on a node URI or on a specific node type. This is
useful when you want to see the impact of the changes made on your
aggregation processors, without having to rescan all sources.
|
Click Expand neighbors
|
- If the selected node has no arcs, it fetches its arcs with a depth =
1, and displays at most the number of arcs specified in Max.
arcs per node.
- If the selected node has some arcs,
it replaces truncated arcs by real arcs for at most the number of arcs
specified in Max. arcs per node.
For example, if a node has 25 arcs, and Max. arcs per
node is 10. When the graph is displayed, only 10 arcs are
displayed for this node, and an extra arc labeled "15 truncated arcs" is
added. To see these 15 hidden arcs, select the node and click
Expand neighbors. 10 extra arcs from the
truncated arcs are added to the graph. The node now has 20 arcs and 5
truncated arcs. Click Expand neighbors again, and
the node have its 25 arcs displayed.
|
Check Document payload
|
See the metas, parts, and directives contained in the document.
|
Check Node arcs
|
See the arcs pointing to the selected node. For each, you can see its
name, its direction (From/
To) and if the node is the
Owner of arc (if not, the arc comes from another
document).
|
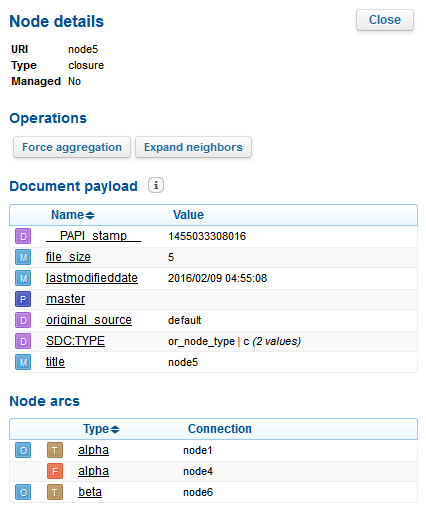
Simulate Matching Elements and Impact Detection
The Simulate tab allows you to test matching rules on a node
to identify which graph elements are impacted. With this tool, you can also check for
impacted nodes, according to existing detected rules, when a change occurs.
-
Choose between the two following modes:
-
Match simulator to enter a matching expression
and see its results on the object graph (see step 4).
-
Impact detection simulator to see the
results of the impact detection for all existing rules present in your
aggregation processors that have already been executed.
-
From Consolidation Server, select the Consolidation
Server instance for which you want to simulate the impact detection an
object graph.
-
In URI(s), select node URIs to simulate the impact
detection starting from these nodes.
-
In Matching expression, enter the matching rule.
-
From Color, you can switch the highlight of either
Nodes or Arcs.
-
Click Submit.
You see the impact of the matching rule on the selected node URIs.
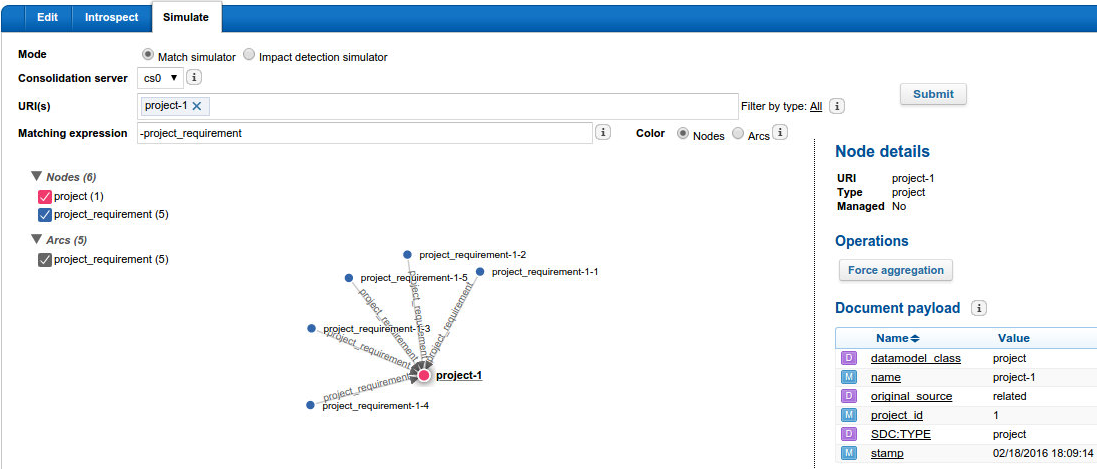
Introspection Client API Usage
Before you begin: The following code snippet shows the java introspection client
used by the Consolidation Introspection Console for the object graph
and document store introspection.
Context:
import com.exalead.consolidationapi.client.answer.Arc;
import com.exalead.consolidationapi.client.answer.Arcs;
import com.exalead.consolidationapi.client.answer.Document;
import com.exalead.consolidationapi.client.answer.DocumentDetails;
import com.exalead.consolidationapi.client.answer.Documents;
import com.exalead.consolidationapi.client.answer.Vertices;
import com.exalead.consolidationapi.client.answer.Type;
import com.exalead.consolidationapi.client.answer.Types;
import com.exalead.consolidationapi.client.query.GetDocumentQuery;
import com.exalead.consolidationapi.client.query.ListArcsQuery;
import com.exalead.consolidationapi.client.query.ListDocumentsQuery;
import com.exalead.consolidationapi.client.query.ListVerticesQuery;
import com.exalead.consolidationapi.client.query.ListTypesQuery;
import com.exalead.consolidationapi.client.answer.Vertex;
/**
* Demonstrate the use of the Consolidation Server introspection client
*/
public class IntrospectionClientDemo {
public static void main(String[] args) {
final IntrospectionClient iC = new IntrospectionClientImpl("localhost", "10553");
// product host name, Consolidation Server monitoring port
try {
// ------------------
// Graph introspection
// ------------------
// List arcs from uris "project-1" & "project-2", using a max exploration depth of 5
final Arcs arcs = iC.listArcs(new ListArcsQuery().withUris("project-1", "project-2")
.withMaxExplorationDepth(5));
for (final Arc arc : arcs) {
System.out.println("Arc: " + arc.getSource() + " -> " + arc.getTarget());
}
// List arc types starting with prefix "rel", and returns only five types
Types types = iC.listArcTypes(new ListTypesQuery("rel").withLimit(5));
for (final Type type : types) {
System.out.println("Arc type starting by 'rel': " + type.getName());
}
// List vertex types starting with prefix "a", and returns an unlimited number of types
types = iC.listVertexTypes(new ListTypesQuery("a").withLimit(0));
for (final Type type : types) {
System.out.println("Vertex type starting by 'a': " + type.getName());
}
// List vertices starting with prefix "a", returns only five nodes
final Vertices nodes = iC.listVertices(new ListVerticesQuery("a").withLimit(5));
for (Vertex vertex : vertices) {
System.out.println("Vertex with a uri starting by 'a' : " + vertex.getUri() + "
[type='" + vertex.getType() + "']");
}
// ------------------
// Storage introspection
// ------------------
// List documents with uri starting with prefix "a", and print details for each
final Documents documents = iC.listDocuments(new ListDocumentsQuery("a"));
for (final Document document : documents) {
System.out.println("Stored document with a uri starting by 'a': " + document.getUri());
System.out.println("Details");
final DocumentDetails details = iC.getDocument(new GetDocumentQuery(document.getUri()));
if (details != null) {
System.out.println("No. of metas: " + details.getMetas().size());
System.out.println("No. of directives: " + details.getDirectives().size());
System.out.println("No. of parts: " + details.getParts().size());
}
}
} catch (final IntrospectionClientException e) {
System.err.println("An error happened during introspection: " + e.getMessage());
}
}
}
Example: My Aggregation Does Not Perform What I Am Expecting
-
Make sure that the objects are correctly connected in the object graph. To do so, use the Consolidation Introspection Console.
-
Then you can look for stack traces in the Consolidation Server logs. You can also modify your transformation and aggregation processors to add logs.
|