ListZonesFromSheet
This function allows you to identifiy which zones are placed on a diagram
view.
Signature
ListZonesFromSheet (iDifSheet, oListOfZonesInfo)
Name |
Input/Output |
Required? |
Type |
Comment |
iDifSheet
|
In |
|
DIFSheet |
The sheet containing the instance of the view
in which the zones are created. |
oListOfZonesInfo
|
Out |
|
List
|
List of zone information objects containing
each zone’s name and description. |
Example
In this example, the functions is applied on a zone called Zone 1.
Let ListOfGBSElements(List)
Let ActiveViewInstance(DIFViewInstance)
Let ActiveSheet(DIFSheet)
Let ListIncludingZonesMessage(String)
GetSchActiveSheetAndView(ActiveSheet, ActiveViewInstance)
if(ActiveSheet <> NULL)
{
//Retrieve List of Including Zones
Let ListOfIncludingZones(List)
ListIncludingZones(ActiveSheet, ”Zone1"), ListOfIncludingZones)
ListIncludingZonesMessage = ListIncludingZonesMessage + " For Zone 1: "
if(ListIncludingZones.Size()>0)
{
//If the Zone is included in one or more others, list them.
let k = 1
let ksize = 0
ksize = ListOfIncludingZones.Size()
for k while k<=ksize
{
Let IncZoneInfo(Feature)
set IncZoneInfo = ListOfIncludingZones.GetItem(k)
ListIncludingZonesMessage = ListIncludingZonesMessage + " " + IncZoneInfo.GetAttributeString("SchZone_Name") + ","
}
ListIncludingZonesMessage = ListIncludingZonesMessage + "|"
}
else
{
ListIncludingZonesMessage = ListIncludingZonesMessage + " NONE " + "|"
}
}
Message(ListIncludingZonesMessage)
ListSpacesFromZones
This function allows you to retrieve the associations between the input zones and the
corresponding space references declared in the platform.
Signature
ListSpacesFromZones (iDifSheet, iListOfZones, oListOfGBSElements)
Name |
Input/Output |
Required? |
Type |
Comment |
iDifSheet
|
In |
|
DIFSheet |
The sheet containing the instance of the view
in which the zones are created. |
iListOfZones
|
In |
|
List
|
List of zone information objects containing
each zone’s name and description. |
oListOfDesignSpaceReference |
Out |
|
List |
List in which each item is a List of
corresponding space references defined in the platform,
associated to the input zones. |
Example
Let ListOfZoneInfos(List)
Let ListOfGBSElements(List)
Let ActiveViewInstance(DIFViewInstance)
Let ActiveSheet(DIFSheet)
Let ListZoneGBSAssociationsMessage(String)
let DSRElement(SSM_DesignSpaceRef)
GetSchActiveSheetAndView(ActiveSheet, ActiveViewInstance)
if(ActiveSheet <> NULL)
{
//Fake Zones for Function demonstration purposes, the best way to retrieve zones is to use ListZonesFromSheet
Let Zone1Info(Feature)
Let Zone2Info(Feature)
Zone1Info.SetAttributeString("SchZone_Name", "Zone 1")
Zone1Info.SetAttributeString("SchZone_Category", "Deck1")
Zone1Info.SetAttributeString("SchZone_Description", "Storage Room 1")
Zone2Info.SetAttributeString("SchZone_Name", "Zone 2")
Zone2Info.SetAttributeString("SchZone_Category", "Deck2")
Zone2Info.SetAttributeString("SchZone_Description", "Storage Room 2")
listOfZoneInfos.Append(Zone1Info)
listOfZoneInfos.Append(Zone2Info)
if(ListOfZoneInfos.Size() > 0)
{
//If Zones Exist, Retrieve their Associated Spaces
ListSpacesFromZones(ActiveSheet, ListOfZoneInfos, ListOfGBSElements)
ListZoneGBSAssociationsMessage = "The Associated GBS Elements are: " + "|"
let j = 1
let jsize = 0
jsize = ListOfZoneInfos.Size()
for j while j<=jsize
{
//Retrieve Zone Attributes
Let ZoneInfo(Feature)
set ZoneInfo = ListOfZoneInfos.GetItem(j)
ListZoneGBSAssociationsMessage = ListZoneGBSAssociationsMessage + " For Zone " + ZoneInfo.GetAttributeString("SchZone_Name") + ": "
//Retrieve associated Space Element Attributes
Let GBSInfoList(List)
set GBSInfoList = ListOfGBSElements.GetItem(j)
if(GBSInfoList<>NULL)
{
let k1 = 1
let ksize1 = 0
ksize1 = GBSInfoList.Size()
if(ksize1>0)
{
for k1 while k1<=ksize1
{
Let GBSInfo(Feature)
set GBSInfo = GBSInfoList.GetItem(k1)
if(GBSInfo <>NULL)
{
set DSRElement = GBSInfo.GetAttributeObject("Space_Ref")
if(DSRElement <> NULL)
ListZoneGBSAssociationsMessage = ListZoneGBSAssociationsMessage + DSRElement.Name + ","
else
ListZoneGBSAssociationsMessage = ListZoneGBSAssociationsMessage + "NULL,"
}
else
ListZoneGBSAssociationsMessage = ListZoneGBSAssociationsMessage + "NULL " + "|"
}
ListZoneGBSAssociationsMessage = ListZoneGBSAssociationsMessage + "|"
}
else
ListZoneGBSAssociationsMessage = ListZoneGBSAssociationsMessage + "NULL " + "|"
}
else
ListZoneGBSAssociationsMessage = ListZoneGBSAssociationsMessage + "NULL " + "|"
}
}
}
Message(ListZoneGBSAssociationsMessage)
ListIncludingZones
This function checks, for a given zone, the zones including this zone.
Signature
ListIncludingZones (iDifSheet, iZoneName, oListOfIncludingZonesInfo)
Name |
Input/Output |
Required? |
Type |
Comment |
iDifSheet
|
In |
|
DIFSheet |
The sheet containing the instance of the view
in which the zones are created. |
iZoneName
|
In |
|
List
|
Zone Name for which you need to know the
including zones encapsulating it. |
oListOfIncludingZonesInfo |
Out |
|
List |
List of zone information objects that hold the information about every
zone that encapsulates the input zone, from closest to the
furthest. |
Example
In the following example, the function is set on a zone called Zone 1
to determine if it is nested in another zone.
Let ListOfGBSElements(List)
Let ActiveViewInstance(DIFViewInstance)
Let ActiveSheet(DIFSheet)
Let ListIncludingZonesMessage(String)
GetSchActiveSheetAndView(ActiveSheet, ActiveViewInstance)
if(ActiveSheet <> NULL)
{
//Retrieve List of Including Zones
Let ListOfIncludingZones(List)
ListIncludingZones(ActiveSheet, ”Zone1"), ListOfIncludingZones)
ListIncludingZonesMessage = ListIncludingZonesMessage + " For Zone 1: "
if(ListIncludingZones.Size()>0)
{
//If the Zone is included in one or more others, list them.
let k = 1
let ksize = 0
ksize = ListOfIncludingZones.Size()
for k while k<=ksize
{
Let IncZoneInfo(Feature)
set IncZoneInfo = ListOfIncludingZones.GetItem(k)
ListIncludingZonesMessage = ListIncludingZonesMessage + " " + IncZoneInfo.GetAttributeString("SchZone_Name") + ","
}
ListIncludingZonesMessage = ListIncludingZonesMessage + "|"
}
else
{
ListIncludingZonesMessage = ListIncludingZonesMessage + " NONE " + "|"
}
}
Message(ListIncludingZonesMessage)
GetSchActiveSheetAndView
This function retrieves the active sheet and the active view
under the active object. The active view is the view that is currently
displayed.
Signature
GetSchActiveSheetAndView(oDifActiveSheet : DIFSheet, oDifActiveViewInstance : DIFViewInstance) : Integer
Arguments
Name |
Input/Output |
Required? |
Type |
Comment |
oDifActiveSheet
|
Out |
|
DIFSheet |
The active sheet |
oDifActiveViewInstance
|
Out |
|
DIFViewInstance |
The active view |
Example
In the following example, this rule sets colors and changes the line type and the
line thickness of all the Equipments represented in the active view.
Let MyInstance(PLMCoreInstance)
Let EquipmentInstance(EnsLogicalEquipmentInst)
Let ListOfSheets(List)
Let ListOfViews(List)
Let MySheet(DifSheet)
Let MyView(DifViewInstance)
Let ListRGB(List)
Let ListGraphAttrNames(List)
Let ListGraphAttrValues(List)
set MyInstance = LogOcc.Instance
set EquipmentInstance = MyInstance
//Only for Equipments
if(EquipmentInstance <> NULL)
{
//Retrieve the active sheet an view
GetSchActiveSheetAndView(MySheet, MyView)
//Add the red color in the graphic attributes list
ListGraphAttrNames->Append("LineColor")
ListRGB->Append(255)
ListRGB->Append(0)
ListRGB->Append(0)
ListGraphAttrValues->Append(ListRGB)
//Change the line type
ListGraphAttrNames->Append("LineType")
ListGraphAttrValues->Append(3)
//Change the line thickness
ListGraphAttrNames->Append("LineThickness")
ListGraphAttrValues->Append(6)
//Set the color on each view of the session
SetSch2DGraphicProperties(MyInstance, MyView, ListGraphAttrNames, ListGraphAttrValues)
}
GetSchSymbolInformations
This function provides information about all the symbols
representing the input instance.
Signature
GetSchSymbolInformations(iPLMInstance : PLMCoreInstance, oListOfSheets : List, oListOfViews : List, oListOfSymbolAttributes : List) : Integer
Arguments
Name |
Input/Output |
Required? |
Type |
Comment |
iPLMInstance
|
In |
|
PLMCoreInstance |
The instance of an object. |
oListOfSheets
|
Out |
|
List
|
The sheets where the input instance is
represented by a symbol. |
oListOfViews
|
Out |
|
List
|
The view instances where the input PLM
instance is represented by a symbol. |
oListOfSymbolAttributes
|
Out |
|
List
|
The list of properties of the symbols
representing the input instance. Each object in this list has:
-
SymbolInstance_Name: the name of
the symbol instance (String)
- SymbolInstance_PositionX_InSheet:
the X coordinate of the position of the symbol in the
sheet (Double)
- SymbolInstance_PositionY_InSheet:
the Y coordinate of the position of the symbol in the
sheet (Double)
- SymbolInstance_PositionX_InFrame:
the X coordinate of the position of the symbol in the
frame (String)
- SymbolInstance_PositionY_InFrame:
the Y coordinate of the position of the symbol in the
frame (String)
- SymbolInstance_Rotation: the
angle rotation of the symbol (Double)
- SymbolInstance_IsFlip: return
true if the symbol is flip else false (Boolean)
- SymbolInstance_Scale: the scale
of the symbol (Double)
- SymbolInstance_ContainerType:
Geographical Container or Composite or Composite
Container or None (String)
- SymbolInstance_ZoneComputationMode:
OneZone or TwoZones (String)
- SymbolInstance_ZoneNamesList: the
list of the zones names containing an existing Symbol
(List of String)
|
Note:
The three output lists must have the same size (mandatory).
Example
Let LogInstance(PLMCoreInstance)
Let ListOfSheets(List)
Let ListOfViews(List)
Let ListOfSymbolInfos(List)
Set LogInstance = ThisObject.Instance
GetSchSymbolInformations(LogInstance, ListOfSheets, ListOfViews, ListOfSymbolInfos)
Let j = 1
Let jsize = 0
Let DisplayMessage(String)
jsize = ListOfSymbolInfos->Size()
if(ListOfSheets->Size() == jsize and ListOfViews->Size() == jsize)
{
for j while j<=jsize
{
set DisplayMessage =""
Let Sheet(DIFSheet)
Let SheetInstance(DIFSheetInstance)
Let View(DIFViewInstance)
Let SymbolInfo(Feature)
Let ListofZone(List)
set Sheet = ListOfSheets.GetItem(j)
set SheetInstance = ListOfSheets.GetItem(j)
set View = ListOfViews.GetItem(j)
set SymbolInfo = ListOfSymbolInfos.GetItem(j)
set ListofZone = SymbolInfo.GetAttributeObject("SymbolInstance_ZoneNamesList")
if((SheetInstance <> NULL or Sheet <> NULL) and View <> NULL and SymbolInfo <> NULL)
{
DisplayMessage = "- Symbol Name: "+ SymbolInfo.GetAttributeString("SymbolInstance_Name") +"|"
DisplayMessage = DisplayMessage + "- Representation Mode : " + SymbolInfo.GetAttributeString("SymbolInstance_RepresentationMode")+ "|"
DisplayMessage = DisplayMessage + "- It is in "
if(Sheet <> NULL)
DisplayMessage = DisplayMessage + Sheet.Name
if(SheetInstance <> NULL)
DisplayMessage = DisplayMessage + SheetInstance.Name
DisplayMessage = DisplayMessage + " : "+ View.Name + "|"
DisplayMessage = DisplayMessage +"- Position in Sheet : (" +SymbolInfo.GetAttributeReal("SymbolInstance_PositionX_InSheet") + ", "+ SymbolInfo.GetAttributeReal("SymbolInstance_PositionY_InSheet") + ") |"
DisplayMessage = DisplayMessage +"- Rotation : " +SymbolInfo.GetAttributeReal("SymbolInstance_Rotation") + " |"
DisplayMessage = DisplayMessage +"- IsFlip : " +SymbolInfo.GetAttributeString("SymbolInstance_IsFlip") + " |"
DisplayMessage = DisplayMessage +"- Scale : " +SymbolInfo.GetAttributeReal("SymbolInstance_Scale") + " |"
DisplayMessage = DisplayMessage +"- Container Type : " +SymbolInfo.GetAttributeString("SymbolInstance_ContainerType") + " |"
DisplayMessage = DisplayMessage +"- Zone Computation Mode : " +SymbolInfo.GetAttributeString("SymbolInstance_ZoneComputationMode") + " |"
DisplayMessage = DisplayMessage +"- Position in Frame : (" +SymbolInfo.GetAttributeString("SymbolInstance_PositionX_InFrame") + ", "+ SymbolInfo.GetAttributeString("SymbolInstance_PositionY_InFrame") + ") |"
DisplayMessage = DisplayMessage +"- In Zones : ("
let ZoneName(string)
for ZoneName inside ListofZone
DisplayMessage = DisplayMessage + ZoneName +", "
DisplayMessage = DisplayMessage +") |"
DisplayMessage = DisplayMessage +"- Father Name : " + SymbolInfo.GetAttributeString("SymbolInstance_FatherContainerSymbolName") + " |"
DisplayMessage = DisplayMessage +"- Father Instance Name : " + SymbolInfo.GetAttributeString("SymbolInstance_FatherContainerInstanceName") + " |"
Message(DisplayMessage)
}
}
}
ListSheetsAndViewsFromObject
This function lets you know in which diagram views a logical
instance is represented.
Signature
ListSheetsAndViewsFromObject(iPLMInstance: PLMCoreInstance, oSheetsList: List, oViewsList: List): Integer
Name |
Input/Output |
Required? |
Type |
Comment |
iPLMInstance
|
In |
|
PLMCoreInstance |
Instance which are represented as a symbol,
route or port and are searched in sheet and diagram views.
|
oSheetsList
|
Out |
|
List
|
List of sheets where the instance is
represented. |
oViewsList
|
Out |
|
List
|
List of diagram view where the instance is
represented. |
Note:
oSheetsList and oViewsList have the same size.
For each diagram view instance in oSheetsList , the
corresponding sheet can be found at the same index in
oViewsList .
ReplaceSymbolInSchematicView
This function lets you switch symbol in a diagram view for a given
logical instance.
Signature
ReplaceSymbolInSchematicView (iPLMInstance: PLMInstance, iSymbolInstanceName: SymbolInstanceName, iSymbolReferenceName: SymbolReferenceName, iDifViewReference: DIFViewReference): Integer
Name |
Input/Output |
Required? |
Type |
Comment |
iPLMInstance
|
In |
|
PLMCoreReference |
The logical instance of the object for which
the symbol is to be replaced. |
iSymbolInstanceName
|
In |
|
String |
The name of the symbol instance representing
the input logical instance. Those names can be retrieved using
the GetSchSymbolInformations method. Note:
- If this input string is empty, all symbol instances
of the input logical instance will be replaced in
the diagram view.
- If this input string is not empty but does not match
any existing symbolinstance representing the input
logical instance, the function will fail.
|
iSymbolReferenceName |
In |
|
String |
The name of a symbol reference associated with
the logical reference of the input logical instance. This name
can be retrieved using theListSymbolReferenceNamesFromPLMReference
method. Note:
If this input string is empty or does not
match any existing symbol reference available for the
input logical instance, the function will
fail.
|
iDifViewReference
|
In |
|
DIFView |
The diagram view in which the symbol is to be
replaced. Note:
If this input is NULL , symbols
will be replaced in every diagram views of the
session.
|
- Return Code
-
Integer
Example
In this first example, the function updates a symbol reference from a diagram
view. .
//Retrieve all the symbols from the input view
let DifViewRef(DifView)
set DifViewRef = DifViewInst.Reference
let ListOfSymbolsInView(List)
DifViewRef.GetSymbols(ListOfSymbolsInView)
Message("Replacing " + ListOfSymbolsInView.Size() + " symbols from " + DifViewRef.Name)
//For each symbol in the view
let SymbolToReplace(DifSymbol)
let DisplayedMessage(String)
for SymbolToReplace inside ListOfSymbolsInView
{
//Retrieve the symbol instance name
let SymbolInstanceName(String)
set SymbolInstanceName = SymbolToReplace.GetAttributeString("alias")
//Retrieve the PLM Instance and PLM Reference represented by the symbol
let RepresentedInstance(RFLVPMLogicalInstance)
let RepresentedReference(PLMCoreReference)
let ListOfReprentedObjects(List)
SymbolToReplace.GetRepresentedObject(ListOfReprentedObjects)
if(ListOfReprentedObjects.Size() > 0)
{
let VODOfRepresentedObject(List)
set VODOfRepresentedObject = ListOfReprentedObjects.GetItem(1)
if(VODOfRepresentedObject.Size() > 0)
{
set RepresentedInstance = VODOfRepresentedObject.GetItem(VODOfRepresentedObject.Size())
set RepresentedReference = RepresentedInstance.Reference
}
}
//Retrieve the names of the Symbol References available for the PLM Reference represented by the symbol
let ListOfSymbolReferencesNames(List)
ListSymbolReferenceNamesFromPLMReference(RepresentedReference, ListOfSymbolReferencesNames)
//Replace the symbol by another (in this example we take the second symbol reference in the list)
let SymbolReferenceName(String)
set SymbolReferenceName = ListOfSymbolReferencesNames.GetItem(2)
ReplaceSymbolInSchematicView(RepresentedInstance, SymbolInstanceName, SymbolReferenceName, DifViewRef)
//Display a message on the screen
set DisplayedMessage = "Symbol " + SymbolInstanceName + " of "+ RepresentedInstance.Name + " are replaced by " + SymbolReferenceName + " from the PLM Reference " + RepresentedReference.Name
Message(DisplayedMessage)
In this second example, the function updates a symbol reference from a logical
instance. //Retrieve the selected PLM Instance and PLM Reference
let RepresentedInstance(PLMCoreInstance)
set RepresentedInstance = PLMOccurrence.Instance
let RepresentedReference(PLMCoreReference)
set RepresentedReference = PLMOccurrence.Reference
//Retrieve the names of the Symbol References available for the PLM Reference represented by the symbol
let ListOfSymbolReferencesNames(List)
ListSymbolReferenceNamesFromPLMReference(RepresentedReference, ListOfSymbolReferencesNames)
//Replace all the symbols of the input PLM Instance by another (in this example we take the second symbol reference in the list)
let SymbolReferenceName(String)
set SymbolReferenceName = ListOfSymbolReferencesNames.GetItem(2)
ReplaceSymbolInSchematicView(RepresentedInstance, "", SymbolReferenceName, NULL)
//Display a message on the screen
let DisplayedMessage(String)
set DisplayedMessage = "Symbols of "+ RepresentedInstance.Name + " are replaced by " + SymbolReferenceName + " from the PLM Reference " + RepresentedReference.Name
Message(DisplayedMessage)
SetSch2DGraphicProperties
This function allows you to modify the graphic properties of
all symbols
Signature
SetSch2DGraphicProperties(iPLMInstance : PLMCoreInstance, iDifViewInstance : DIFViewInstance, iListOfParametersNames : List, iListOfParametersValues : List) : Integer
Arguments
Name |
Input/Output |
Required? |
Type |
Comment |
iPLMInstance
|
In |
|
PLMCoreInstance |
The instance of the object represented by the
symbol, route or port to modify. |
iDifViewInstance
|
In |
|
DIFViewInstance |
The view where the input instance is
represented. |
iListOfParametersNames
|
In |
|
List
|
The list of graphic properties names to modify.
The accepted values are:
- LineColor
- LineType
- LineThickness
|
iListOfParametersValues
|
In |
|
List
|
The list of graphic properties values to set
on the symbol to modify.
- LineColor values are a list of
integer between 0 and 255. The first integer is the red
component, the second is the green component and the
last is the blue component.
- LineType values are integers.
- LineThickness values are
integers.
|
Example
Let MyInstance(PLMCoreInstance)
Let EquipmentInstance(EnsLogicalEquipmentInst)
Let ListOfSheets(List)
Let ListOfViews(List)
Let MySheet(DifSheet)
Let MyView(DifViewInstance)
Let ListRGB(List)
Let ListGraphAttrNames(List)
Let ListGraphAttrValues(List)
set MyInstance = LogOcc.Instance
set EquipmentInstance = MyInstance
//Only for Equipments
if(EquipmentInstance <> NULL)
{
//Retrieve the sheets an views where the instance is represented
ListSheetsAndViewsFromObject(MyInstance, ListOfSheets, ListOfViews)
//Add the red color in the graphic attributes list
ListGraphAttrNames->Append("LineColor")
ListRGB->Append(255)
ListRGB->Append(0)
ListRGB->Append(0)
ListGraphAttrValues->Append(ListRGB)
//Change the line type
ListGraphAttrNames->Append("LineType")
ListGraphAttrValues->Append(3)
//Change the line thickness
ListGraphAttrNames->Append("LineThickness")
ListGraphAttrValues->Append(6)
//Set the color on each view of the session
for MyView inside ListOfViews
{
SetSch2DGraphicProperties(MyInstance, MyView, ListGraphAttrNames, ListGraphAttrValues)
}
}
GetSchematicRoutedLengthPerView
This function allows the length of logical pipe to be
retrieved.
Signature
GetSchematicRoutedLengthPerView(iPLMInstance : PLMCoreInstance, oListOfRoutedLength : List, oListOfViewInstance : List, oListOfOnOffSheetConnectors : List) : Integer
Arguments
Name |
Input/Output |
Required? |
Type |
Comment |
iPLMInstance
|
In |
|
PLMCoreInstance |
- |
oListOfRoutedLength
|
Out |
|
List
|
Lists the total length of all routes
representing the given logical instance per view and in
millimeter (sheet size). |
oListOfViewInstance
|
Out |
|
List
|
Lists view instances. |
oListOfOnOffSheetConnectors
|
Out |
|
List
|
Lists Boolean. If the Boolean is TRUE, it
means the route is connected to a sheet connector. If the
Boolean is FALSE, it means the route is not connected to a sheet
connector. |
Example
This script prompt a message showing the pipe instance name, the
length in each view where it is placed and the presence or not of a sheet
connector.
Let ListOfRoutedLength(List)
Let ListOfViewInstances(List)
Let ListOfOnOffSheetConnectors(List)
Let PipeInstance(PLMCoreInstance)
Let j = 1
Let jsize = 0
Let RoutedLength(Real)
Let DisplayMessage(String)
Let OnOffSheetConnector(Boolean)
Let DifViewInstance(DIFViewInstance)
Set PipeInstance = ThisObject.Instance
GetSchematicRoutedLengthPerView(PipeInstance, ListOfRoutedLength, ListOfViewInstances, ListOfOnOffSheetConnectors)
jsize = ListOfRoutedLength->Size()
if(ListOfViewInstances->Size() == jsize and ListOfOnOffSheetConnectors->Size() == jsize)
{
DisplayMessage = PipeInstance.GetAttributeString("PLM_ExternalID")
DisplayMessage = DisplayMessage + " = "
for j while j<=jsize
{
set DifViewInstance = ListOfViewInstances[j]
set RoutedLength = ListOfRoutedLength[j]
set OnOffSheetConnector = ListOfOnOffSheetConnectors[j]
DisplayMessage = DisplayMessage + RoutedLength + " mm in " + DifViewInstance.GetAttributeString("PLM_ExternalID")
if(OnOffSheetConnector == TRUE)
DisplayMessage = DisplayMessage + " and has an On/Off sheet connector;"
else
DisplayMessage = DisplayMessage + " and does not have an On/Off sheet connector;"
}
Message(DisplayMessage)
ListSheetsFromObject
This function lists all the sheets where a given instance is
represented.
Signature
ListSheetsFromObject(iPLMInstance : PLMCoreInstance, oListOfSheets : List) : Integer
Arguments
Name
|
Input/Output
|
Required?
|
Type
|
Comment
|
iPLMInstance
|
In
|
|
PLMCoreInstance
|
-
|
oListOfSheets
|
Out
|
|
List
|
Lists the views containing the instance.
|
Example
This script prompt a message showing the instance name and where it
is placed.
Let LogInstance(PLMCoreInstance)
Let ListOfSheets(List)
Set LogInstance = ThisObject.Instance
ListSheetsFromObject(LogInstance, ListOfSheets)
Let j = 1
Let jsize = ListOfSheets->Size()
Let DisplayMessage(String)
if(jsize > 0 and LogInstance <> NULL)
{
for j while j<=jsize
{
set DisplayMessage =""
Let Sheet(DIFSheet)
set Sheet = ListOfSheets.GetItem(j)
if(Sheet <> NULL )
{
DisplayMessage = LogInstance.Name
DisplayMessage = DisplayMessage + " is in "
DisplayMessage = DisplayMessage + Sheet.Name
Message(DisplayMessage)
}
}
}
GetSchSheetConnectorInformations
This function allows you to retrieve information about connected sheet
connectors placed in different
sheets.
Signature
CATGetSchSheetConnectorInformations(iRoutableInstance : PLMCoreInstance, oListOfSheetCntrInfos : List) : Integer
Arguments
Name |
Input/Output |
Required? |
Type |
Comment |
iRoutableInstance
|
In |
|
PLMCoreInstance |
The logical instance of the routable. |
oListOfSheetCntrInfos
|
Out |
|
List
|
Lists of properties of the sheet connector
connected to the input instance. Each object in the list has
the following string attributes:
- SheetConnector_Object_Name:
the name of the analyzed sheet connector instance
(String)
- SheetConnector_RelatedOffSheetObject_Name:
the list of names of the offsheets related to the
sheet connector (List of String)
- SheetConnector_RelatedRoutable:
the routable related to this offsheet on the other
side (CATIPLMComponent)
- SheetConnector_RelatedComponent:
the component related to this offsheet on the other
side (CATIPLMComponent)
- SheetConnectors_SheetReference:
the sheet reference of this sheet connector
(CATBaseUnknown)
- SheetConnector_SheetInstance:
the sheet instance of this sheet connector
(CATBaseUnknown)
- SheetConnector_ViewReference:
the view reference of this sheet connector
(CATBaseUnknown)
- SheetConnector_ViewInstance:
the view instance of this sheet connector
(CATBaseUnknown)
- SheetConnector_PositionX_InSheet:
the X coordinate of the symbol's position in the
sheet (Double)
- SheetConnector_PositionY_InSheet:
the Y coordinate of the symbol's position in the
sheet (Double)
- SheetConnector_FramePositionX:
the X coordinate of the symbol's position in the
frame (String)
- SheetConnector_FramePositionY:
the Y coordinate of the symbol's position in the
frame (String)
|
Example
Two sheets are created in two different diagram views containing a routable connected to two
different sheet connectors.
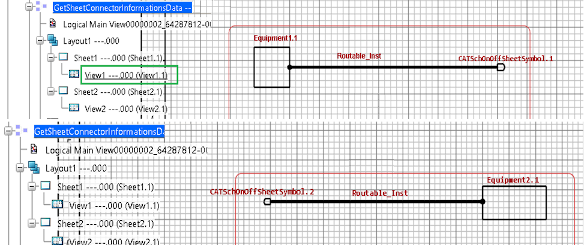
In the following example, the function is set for:
- A routable connected to two different sheet connectors
- Two routables connected to two different sheet connectors
- A routable connected to an unconnected sheet connector
Let RoutableInstance(PLMCoreInstance)
Let DisplayMessage(String)
Set RoutableInstance = ThisObject.Instance
Let ListOfSheetCntrInfos(List)
GetSchSheetConnectorInformations(RoutableInstance, ListOfSheetCntrInfos)
Let j = 1
Let jsize = 0
jsize = ListOfSheetCntrInfos->Size()
Let ListofRelatedName(List)
for j while j<=jsize
{
Let SheetCntrInfos(Feature)
set SheetCntrInfos = ListOfSheetCntrInfos.GetItem(j)
if(SheetCntrInfos <> NULL)
{
//Retrieve the information about each sheet connector
DisplayMessage = " Input Routable : " + RoutableInstance.GetAttributeString("PLM_ExternalID") + " | "
DisplayMessage = DisplayMessage + "Active Sheet Connector Name = " + SheetCntrInfos.GetAttributeString("SheetConnector_Object_Name")+ " | "
Let RelatedOffSheetName(String)
Let i = 1
Set ListofRelatedName = SheetCntrInfos.GetAttributeObject("SheetConnector_RelatedOffSheetObject_Name")
for i while i<=ListofRelatedName->Size()
{
RelatedOffSheetName = ListofRelatedName[i]
if (RelatedOffSheetName <> "")
DisplayMessage = DisplayMessage + " | " + "Related OffSheet Name= " + RelatedOffSheetName+ " | "
}
Let RelatedRoutableInstance(PLMCoreInstance)
Set RelatedRoutableInstance = SheetCntrInfos.GetAttributeObject("SheetConnector_RelatedRoutable")
if (RelatedRoutableInstance <> NULL)
DisplayMessage = DisplayMessage + "Related Routable Instance = "+ RelatedRoutableInstance.GetAttributeString("PLM_ExternalID")+ " | "
Let RelatedComponentInstance(PLMCoreInstance)
Set RelatedComponentInstance = SheetCntrInfos.GetAttributeObject("SheetConnector_RelatedComponent")
if (RelatedComponentInstance <> NULL)
DisplayMessage = DisplayMessage + "Related Component Instance = "+ RelatedComponentInstance.GetAttributeString("PLM_ExternalID")+ " | "
Let SheetInst(DIFSheetInstance)
Set SheetInst = SheetCntrInfos.GetAttributeObject("SheetConnector_SheetInstance")
if (SheetInst <> NULL)
DisplayMessage = DisplayMessage + " | " + "Sheet Instance = " + SheetInst.GetAttributeString("PLM_ExternalID")
Let Sheet(DIFSheet)
Set Sheet = SheetCntrInfos.GetAttributeObject("SheetConnector_SheetReference")
if (Sheet <> NULL)
DisplayMessage = DisplayMessage + " | " + "Sheet Reference = " + Sheet.Name+ " | "
Let ViewInstance(DIFViewInstance)
Set ViewInstance = SheetCntrInfos.GetAttributeObject("SheetConnector_ViewInstance")
DisplayMessage = DisplayMessage + "View Instance= " + ViewInstance.GetAttributeString("PLM_ExternalID")+ " | "
Let ViewRef(DIFView)
Set ViewRef = SheetCntrInfos.GetAttributeObject("SheetConnector_ViewReference")
DisplayMessage = DisplayMessage + "View Reference= " + ViewRef.Name+ " | "
Let PositionX(Real)
Set PositionX = SheetCntrInfos.GetAttributeReal("SheetConnector_PositionX_InSheet")
DisplayMessage = DisplayMessage + " | "+ "PositionX= " + PositionX+ " | "
Let PositionY(Real)
Set PositionY = SheetCntrInfos.GetAttributeReal("SheetConnector_PositionY_InSheet")
DisplayMessage = DisplayMessage+ "PositionY= " + PositionY+ " | "
Let FramePositionX(String)
Set FramePositionX = SheetCntrInfos.GetAttributeString("SheetConnector_FramePositionX")
DisplayMessage = DisplayMessage + "FramePositionX= " + FramePositionX+ " | "
Let FramePositionY(String)
Set FramePositionY = SheetCntrInfos.GetAttributeString("SheetConnector_FramePositionY")
DisplayMessage = DisplayMessage + "FramePositionY= " + FramePositionY+ " | "
Message(DisplayMessage)
}
}
Results
For the diagram view containing a routable connected to two different sheet
connectors, the previous business rule returns the following information
Input |
Name |
Input Routable |
Routable_Inst |
Output |
Properties |
Active Sheet Connector Name |
CATSchOnOffSheetSymbol.1 |
Related OffSheet Name |
CATSchOnOffSheetSymbol.2 |
Related Routable Instance |
Routable_Inst |
Related Component Instance |
Equipement2.1 |
Sheet Instance |
Sheet1.1 |
Sheet Reference |
Sheet1 |
View Instance |
View1.1 |
View Reference |
View1 |
Position X |
606.3 |
Position Y |
640 |
FramePosition X |
C |
FramePosition Y |
4 |
Output |
Properties |
Active Sheet Connector Name |
CATSchOnOffSheetSymbol.2 |
Related OffSheet Name |
CATSchOnOffSheetSymbol.1 |
Related Routable Instance |
Routable_Inst |
Related Component Instance |
Equipement1.1 |
Sheet Instance |
Sheet2.1 |
Sheet Reference |
Sheet2 |
View Instance |
View2.1 |
View Reference |
View2 |
Position X |
393.7 |
Position Y |
630 |
FramePosition X |
B |
FramePosition Y |
4 |
ListSymbolReferenceNamesFromPLMReference
This function lets you retrieve the names of the symbol references
associated to a given logical
reference.
Signature
ListSymbolReferenceNamesFromPLMReference(iPLMReference : PLMReference, PLMCoreReference : PLMCoreReference) : Integer
Name |
Input/Output |
Required |
Type |
Comment |
iPLMReference |
In |
|
PLMCoreReference |
The logical reference of the object for which the symbol is to be
replaced. |
oListOfSymbolRefNames |
Out |
|
List
|
The list of symbol references names associated to the input logical
reference. |
|