new
Function used to deal with the instantiation of children in a list.
Signaturenew(concept: String, name: String,
father: BaseConcept, [ freeArguments: ObjectType, ..]) : UndefinedType
Arguments
Name | Input / Output | Required? | Type | Comment |
concept | In | Yes | String | Name of the concept to be instantiated. |
name | In | Yes | String | Name of the object to be created. If the object is instantiated below another object, the name of the created object must be the name of the child attribute for which it is created. |
father | In | Yes | BaseConcept | None if root object otherwise, the father. |
freeArguments | In | No | ObjectType | Used to valuate the concept inputs. Arguments must be entered following the order defined on the concept. |
Example
Concept Adder : BaseConcept
{
Object: VoidType;
Attributes
{
Inputs
{
Integer a;
Integer b;
}
Outputs
{
Integer result = a+b;
}
}
}
Concept AdderOfAdder : BaseConcept
{
Object : VoidType;
Attributes
{
Inputs
{
Integer in1;
Integer in2;
Integer in3;
Integer in4;
}
Outputs
{
Integer outAdder;
}
}
Children
{
Adder firstAdder;
Adder secondAdder;
}
Rules
{
Rule
{
/* First case, finalize is done automatically by "new"
because all inputs are valuated within the free arguments */
firstAdder = new("Adder","firstAdder",this,in1,in2)
secondAdder = new("Adder","secondAdder",this)
/* No input is given within the free arguments */
secondAdder.a = in3
secondAdder.b = in4
secondAdder.Finalize() /* Necessary to compute the result if we
want to access it in this rule */
outAdder = firstAdder.result + secondAdder.result
}
}
}
newInList
Function used to deal with the instantiation of children in a list.
SignaturenewInList(concept: String, name: String,
father: BaseConcept, list: List [, freeArguments:
ObjectType, ..]) : UndefinedType
Arguments
Name | Input / Output | Required? | Type | Comment |
concept | In | Yes | String | Name of the concept to be instantiated. |
name | In | Yes | String | Name of the object to be created. |
father | In | Yes | BaseConcept | None if root object otherwise, the father. |
list | Out | Yes | List | When a List child is defined in a concept, newInList enables you to create the child and to insert it into the list. |
freeArguments | In | No | ObjectType | Used to valuate the concept inputs. Arguments must be entered following the order defined on the concept. |
Example
Concept MyListConcept : KBEFeature
{
Children
{
List(MyChildConcept) lChild1;
}
Rules
{
Rule
{
let i(Integer)
let currentChild(MyChildConcept)
i = 0
for i while i <= 10
{
currentChild = newInList("MyChildConcept","child" + i, this,lChild1,i)
}
};
}
}
Concept MyChildConcept : BaseConcept
{
Attributes
{
Inputs
{
Integer a;
}
Outputs
{
Integer b;
}
}
} Note:
In the example above, at the end of the evaluation of the Rule in MyListConcept, the lChild1 list will contain 10 objects of MyChildConcept type. From the engine point of view the rule defined in MyListConcept is seen as an evaluator for lChild1.
GetSubConcepts
Function used to return a list containing the name of the sub-concepts of the concept entered in input. The Boolean lets you indicate if you want to get direct sub-concepts only or if you want to recursively get sub-concepts, sub-concepts sub-concepts […]. It allows the user to choose the concept to be used at instantiation.
Arguments
Name | Input / Output | Required? | Type | Comment |
iConceptName | In | Yes | String | Concept name. |
iRecursively | In | Yes | Boolean | If set to true, the recursive mode is enabled. |
ExampleConcept ArithmeticOperationFactory : BaseConcept
{
Object : VoidType;
Attributes
{
Inputs
{
String typeOfOperation;
}
}
Children
{
ArithmeticOperation oper = new(typeOfOperation,"oper",this);
}
Rules
{
Rule on Init
{
typeOfOperation.AuthorizedValues = GetSubConcepts("ArithmeticOperation",TRUE)
}
}
}
Concept ArithmeticOperation : BaseConcept
{
Object : VoidType;
Attributes
{
Inputs
{
Integer a;
Integer b;
}
Outputs
{
Integer result;
}
}
Rules
{
Rule on valuation result
{
Message("result is #",result)
}
}
}
Concept Addition : ArithmeticOperation
{ Rules
{
Rule
{
result a + b
}
}
}
Concept Substraction : ArithmeticOperation
{
Rules
{
Rule
{
result a - b
}
}
}
Concept Multiplication : ArithmeticOperation
{
Rules
{
Rule
{
result a * b
}
}
} When instantiating the ArithmeticOperationFactory concept, you can choose one of the three values for typeOfOperation:
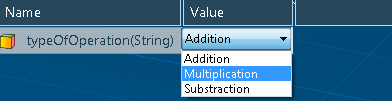
SetWorkingContext
Function used to change the creation container of GSD Features.
SignatureSetWorkingContext(iNewContext: Feature)
Arguments
Name | Input / Output | Required? | Type | Comment |
iNewContext | In | Yes | Feature | |
Example
Concept MyDistanceComputer : BaseConcept
{
Object:VoidType;
Attributes
{
Inputs
{
Point a;
Point b;
BodyFeature context;
}
Outputs
{
Length result;
}
}
Rules
{
Rule
{
let l(Line)
SetWorkingContext(context)
l=line(a,b)
result = length(l)
}
}
} Note:
In the above example, the line (a,b) function is used. This function needs a context to build the line and compute the length. When working with KML, the context cannot be automatically deduced, it must be indicated so that the line function works properly. In this example, the context is the body containing the points a and b.
|