Arguments
Name |
Type |
Description |
iPilotPart |
Feature |
The initial pilot part feature. |
iListPilotFaceContactKeys |
List |
The list of keys of the pilot part's face which is in contact with the joined
part in two dimensions.
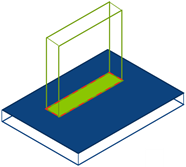
|
iListPilotEdgeContactKeys |
List |
The list of keys of the pilot part's face which is in contact with the joined
part in one dimension.
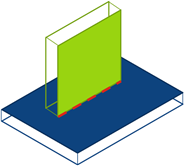
|
iJoinedPart |
Feature |
The initial joined part feature. |
iListJoinedFaceContactKeys |
List |
The list of keys of the joined part's face which is in contact with the pilot
part in two dimensions. |
iListJoinedEdgeContactKeys |
List |
The list of keys of the joined part's face which is in contact with the pilot
part in one dimension. |
iListTemplate |
List |
The list of templates from the structure fastener catalog or library. |
iInterferenceClash |
Boolean |
True for clash. False for contact. |
oNeedSwap |
Boolean |
True if swapping is needed for the pilot and joined parts. False if swapping
is not needed. |
oIndexOfTemplate |
Integer |
Index of the template for the computed pair. |
Sample
/*
* iPilotPart : Feature
* iListPilotFaceContactKeys : List
* iListPilotEdgeContactKeys : List
* iJoinedPart : Feature
* iListJoinedFaceContactKeys : List
* iListJoinedEdgeContactKeys : List
* iListTemplate : List
* iInterferenceClash : Boolean
* oNeedSwap : Boolean
* oIndexOfTemplate : Integer
*/
//For Pilot and Joined part type detection
let PilotPlate(SddPlate)
let PilotSplitPlate(SddSplitPlate)
let PilotContourBased(SddContourBased)
let PilotProfile(SddProfile)
let JoinedPlate(SddPlate)
let JoinedSplitPlate(SddSplitPlate)
let JoinedContourBased(SddContourBased)
let JoinedProfile(SddProfile)
set PilotPlate = iPilotPart
set PilotSplitPlate = iPilotPart
set PilotContourBased = iPilotPart
set PilotProfile = iPilotPart
set JoinedPlate = iJoinedPart
set JoinedSplitPlate = iJoinedPart
set JoinedContourBased = iJoinedPart
set JoinedProfile = iJoinedPart
let PilotType(string)
let JoinedType(string)
if(PilotPlate <> NULL or PilotSplitPlate <> NULL or PilotContourBased <> NULL){
PilotType = "Plate"
}
else if(PilotProfile <> NULL){
PilotType = "Profile"
}
else{
PilotType = "Unknown"
}
if(JoinedPlate <> NULL or JoinedSplitPlate <> NULL or JoinedContourBased <> NULL){
JoinedType = "Plate"
}
else if(JoinedProfile <> NULL){
JoinedType = "Profile"
}
else{
JoinedType = "Unknown"
}
//Create String to check combined type
let CombinedType(string)
CombinedType = PilotType + JoinedType
//Useful keys to computation
let TopBottomFaceKeys(List)
TopBottomFaceKeys.Append("1")
TopBottomFaceKeys.Append("2")
let SideFaceKeys(List)
SideFaceKeys.Append("0")
let StartEndFaceKeys(List)
StartEndFaceKeys.Append("1")
StartEndFaceKeys.Append("2")
let FlangeFaceKeys(List)
FlangeFaceKeys.Append("22")
FlangeFaceKeys.Append("3")
let WebFaceKeys(List)
WebFaceKeys.Append("12")
WebFaceKeys.Append("13")
//Plate-Plate case computation
if(CombinedType == "PlatePlate"){
let IsPilotContactTB(List)
let IsJoinedContactTB(List)
IsPilotContactTB = iListPilotFaceContactKeys.Intersect(TopBottomFaceKeys)
IsJoinedContactTB = iListJoinedFaceContactKeys.Intersect(TopBottomFaceKeys)
let IsPilotEdgeContactTB(List)
let IsPilotEdgeContactSide(List)
let IsJoinedEdgeContactTB(List)
let IsJoinedEdgeContactSide(List)
IsPilotEdgeContactTB = iListPilotEdgeContactKeys.Intersect(TopBottomFaceKeys)
IsPilotEdgeContactSide = iListPilotEdgeContactKeys.Intersect(SideFaceKeys)
IsJoinedEdgeContactTB = iListJoinedEdgeContactKeys.Intersect(TopBottomFaceKeys)
IsJoinedEdgeContactSide = iListJoinedEdgeContactKeys.Intersect(SideFaceKeys)
if(iInterferenceClash == True){
//In case of Clash model
//TODO: It is necessary to write the code in the below to choose template based on geometry computation
}
else if(IsPilotContactTB.Size() > 0 and IsJoinedContactTB.Size() > 0){
//In case of Top and Bottom faces contact, the LID template selected by rule
oIndexOfTemplate = 6
}
else if(IsPilotContactTB.Size() > 0 or IsJoinedContactTB.Size() > 0){
//When Top/Bottom face contact in one part, the TEE template selected by rule
oIndexOfTemplate = 5
if(IsJoinedContactTB.Size() > 0)
oNeedSwap = True
}
else if(iListPilotFaceContactKeys.Size() > 0 or iListJoinedFaceContactKeys.Size() > 0){
//When Top/Bottom faces are not contacted in dimension 2 but side face is then
//Butt template selected by rule
oIndexOfTemplate = 4
}
else{
//No dimension 2 contact between the objects
if(IsPilotEdgeContactTB.Size() == 2 and IsJoinedEdgeContactTB.Size() == 2){
//Both Top and Bottom faces are contacted with other object.
//It is Impossible case.
}
else if((IsPilotEdgeContactTB.Size() == 2 and IsJoinedEdgeContactTB.Size() > 0) or (IsJoinedEdgeContactTB.Size() == 2 and IsPilotEdgeContactTB.Size() > 0)){
//In one part, Top and Bottom faces contact to other.
//On the other part, Top or Bottom face contact to other.
//Then TEE template selected by rule
// ||
// ||
// ======
oIndexOfTemplate = 5
if(IsJoinedEdgeContactTB.Size() == 2)
oNeedSwap = True
}
else{
if(IsPilotEdgeContactTB.Size() == 0 or IsJoinedEdgeContactTB.Size() == 0){
//When there is no contact more than one dimension
//PointConnection template is selected by rule
oIndexOfTemplate = 18
}
else if(IsPilotEdgeContactSide.Size() > 0 or IsJoinedEdgeContactSide.Size() > 0){
//When there is more than one edge connection,
//TEE template is selected by rule
oIndexOfTemplate = 5
}
}
}
}
//Plate-Profile case computation
else if(CombinedType == "PlateProfile" or CombinedType == "ProfilePlate"){
let PlateFaceKey(List)
let PlateEdgeKey(List)
let ProfileFaceKey(List)
let ProfileEdgeKey(List)
let PlateFeature(Feature)
let ProfileFeature(Feature)
if(CombinedType == "PlateProfile"){
PlateFaceKey = iListPilotFaceContactKeys
PlateEdgeKey = iListPilotEdgeContactKeys
PlateFeature = iPilotPart
ProfileFaceKey = iListJoinedFaceContactKeys
ProfileEdgeKey = iListJoinedEdgeContactKeys
ProfileFeature = iJoinedPart
oNeedSwap = True
}
else{
PlateFaceKey = iListJoinedFaceContactKeys
PlateEdgeKey = iListJoinedEdgeContactKeys
PlateFeature = iJoinedPart
ProfileFaceKey = iListPilotFaceContactKeys
ProfileEdgeKey = iListPilotEdgeContactKeys
ProfileFeature = iPilotPart
}
//Check Slot connection
let PanelObj(SddPanel)
let PlateObj(SddPlate)
set PlateObj = PlateFeature
let testSplitPlate(SddSplitPlate)
set testSplitPlate = PlateFeature
if(testSplitPlate <> NULL){ //Get Panel feature from Split Plate
let PLMOwner(VPMRepReference)
PLMOwner = GetPLMOwner(testSplitPlate)
PanelObj = PLMOwner->Find("SddPanel", "", TRUE)
}
let SlotList(List)
if(PanelObj <> NULL){
PanelObj->GetSlots(SlotList)
}
if(PlateObj <> NULL){
PlateObj->GetSlots(SlotList)
}
let IsSlotConnected(Boolean)
IsSlotConnected = FALSE
let SlotFeature(SddSlotPanel)
for SlotFeature inside SlotList{
let PenetratingProfile(Feature)
SlotFeature->GetPenetratingProfile( PenetratingProfile)
if(PenetratingProfile == ProfileFeature){
IsSlotConnected = True
}
}
let IsPlateTB(List) //Plate Top or Bottom Contact
let IsPlateSide(List) //Plate Side contact
IsPlateTB = PlateFaceKey.Intersect(TopBottomFaceKeys)
IsPlateSide = PlateFaceKey.Intersect(SideFaceKeys)
let IsPlateEdgeTB(List) //Plate Top or Bottom Contact
let IsPlateEdgeSide(List) //Plate Side contact
IsPlateEdgeTB = PlateEdgeKey.Intersect(TopBottomFaceKeys)
IsPlateEdgeSide = PlateEdgeKey.Intersect(SideFaceKeys)
let IsProfileSE(List) // Profile Start End Contact
let IsProfileWeb(List) // Profile Web Contact
let IsProfileWebEdge(List) //Profile Web Edge Contact
let IsProfileFlange(List) //Profile Flange Contact
IsProfileSE = ProfileFaceKey.Intersect(StartEndFaceKeys)
IsProfileWeb = ProfileFaceKey.Intersect(WebFaceKeys)
IsProfileWebEdge = ProfileEdgeKey.Intersect(WebFaceKeys)
IsProfileFlange = ProfileFaceKey.Intersect(FlangeFaceKeys)
if(iInterferenceClash == True){
//In case of Clash model
if( IsSlotConnected == True){
//In case of slot connection, the slot template is selected by rule
oIndexOfTemplate = 13
}
//TODO: It is necessary to write the code in the below to choose template based on geometry computation
}
else if( IsSlotConnected == True){
//In case of slot connection, the slot template is selected by rule
oIndexOfTemplate = 13
}
else if( PilotContourBased <> NULL or JoinedContourBased <> NULL){
//In case of bracket contacted, the bracket template is selected by rule
oIndexOfTemplate = 9
}
else if((iListPilotFaceContactKeys.Size() == 0 and iListPilotEdgeContactKeys.Size() == 0) or
(iListJoinedFaceContactKeys.Size() == 0 and iListJoinedEdgeContactKeys.Size() == 0)){
//When there is no contact more than one dimension
//Point connection Template is selected by rule
oIndexOfTemplate = 18
}
else if(IsPlateTB.Size() > 0){
//When profile located on top of Top/Bottom face of the plate
if(IsProfileSE.Size() > 0){
//Profile Start/End face contact plate
//In this case, BUTT template is selected by rule
oIndexOfTemplate = 12
}
else if(IsProfileWeb.Size() > 0){
//Profile Web face contact plate in dimension 2
//In this case, LID template is selected by rule
oIndexOfTemplate = 7
}
else if(IsProfileWebEdge.Size() > 0){
//Profile web face contact plate in dimension 1
//In this case, TEE template is selected by rule
oIndexOfTemplate = 8
}
else if(IsProfileFlange.Size() > 0){
//Profile flange face contact plate in dimension 2
//In this case, LID template is selected by rule
oIndexOfTemplate = 7
}
}
else if(IsPlateSide.Size() > 0){
//When profile located on the side of the plate
if(IsProfileWeb.Size() > 0){
//When web face of the profile contact plate in dimension 2
//in this case, Choke template is selected by rule
oIndexOfTemplate = 11
}
else if(IsProfileWebEdge.Size() > 0){
//When Web face of the profile contact plate in dimension 1
//In this case, Butt template is selected by rule
oIndexOfTemplate = 12
}
else if(IsProfileFlange.Size() > 0){
//When flange face of the profile contact plate in dimension 2
//In this case, Tee template is selected by rule
oIndexOfTemplate = 8
}
else if(IsProfileSE.Size() > 0){
//When Start or end face of the plate contact plate in dimension 2
//In this case, WebFrame template is selected by rule
oIndexOfTemplate = 10
}
}
else{
//When profile and plate does not contact in dimension 2
if(IsPlateEdgeTB.Size() == 2){
//When Plate top and bottom face contact profile in dimension 1
//In this case, TEE template is selected by rule.
oIndexOfTemplate = 8
}
else if(IsPlateEdgeTB.Size() == 1){
//When Plate top or bottom face contact profile in dimension 1
//In this case, Butt template is selected by rule
oIndexOfTemplate = 12
}
else{
//There is no contact more than 1 dimension by plate.
//In this case, PointConnection template is selected by rule
oIndexOfTemplate = 18
}
}
}
//Profile - Profile case computation
else if(CombinedType == "ProfileProfile"){
let IsPSE(List) //Pilot Start End
let IsJSE(List) // Joined Start End
IsPSE = iListPilotFaceContactKeys.Intersect(StartEndFaceKeys)
IsJSE = iListJoinedFaceContactKeys.Intersect(StartEndFaceKeys)
let IsPWF(List) //Pilot Web Face
let IsJWF(List) //Joined Web Face
IsPWF = iListPilotFaceContactKeys.Intersect(WebFaceKeys)
IsJWF = iListJoinedFaceContactKeys.Intersect(WebFaceKeys)
let IsPWE(List) //Pilot Web Edge
let IsJWE(List) //Joined Web Edge
IsPWE = iListPilotEdgeContactKeys.Intersect(WebFaceKeys)
IsJWE = iListJoinedEdgeContactKeys.Intersect(WebFaceKeys)
let IsPF(List) // Pilot Flange
let IsJF(List) // Joined Flange
IsPF = iListPilotFaceContactKeys.Intersect(FlangeFaceKeys)
IsJF = iListJoinedFaceContactKeys.Intersect(FlangeFaceKeys)
//Check slot connection
let SlotConnection(Boolean)
SlotConnection = FALSE
let PilotSlotList(List)
PilotProfile->GetSlots(PilotSlotList)
let JoinedSlotList(List)
JoinedProfile->GetSlots(JoinedSlotList)
let SlotFeature(SddOpening)
for SlotFeature inside PilotSlotList{
let PenetratingFeature(Feature)
SlotFeature.GetPenetratingProfile(PenetratingFeature)
if(PenetratingFeature == JoinedProfile){
SlotConnection = TRUE
oNeedSwap = TRUE
}
}
for SlotFeature inside JoinedSlotList{
let PenetratingFeature(Feature)
SlotFeature.GetPenetratingProfile(PenetratingFeature)
if(PenetratingFeature == PilotProfile){
SlotConnection = TRUE
}
}
if(iInterferenceClash == True){
//In case of Clash model
if(SlotConnection == True){
//When there is a slot connection between pilot and joined part,
//The slot template is selected by rule
oIndexOfTemplate = 16
}
//TODO: It is necessary to write the code in the below to choose template based on geometry computation
}
else if((iListPilotFaceContactKeys.Size() == 0 and iListPilotEdgeContactKeys.Size() == 0) or
(iListJoinedFaceContactKeys.Size() == 0 and iListJoinedEdgeContactKeys.Size() == 0)){
//When there is no contact more than 1 dimension,
//the PointConnection template is selected by rule
oIndexOfTemplate = 18
}
else if(SlotConnection == True){
oIndexOfTemplate = 16
}
else if(IsPSE.Size() > 0 and IsJSE.Size() > 0){
//when Pilot and Joined Extremities contact
//Butt template is selected by rule
oIndexOfTemplate = 15
}
else if(IsPF.Size() > 0 and IsJF.Size() > 0){
//Pilot and Joined Flange contact
//Back to Back template is selected by rule
oIndexOfTemplate = 17
}
else{
//Else cases, TEE template is selected by rule
oIndexOfTemplate = 14
}
}