Connect Employees to Services and Services to Companies
We want to connect each employee to the service, and the service to the appropriate company with the following data model.
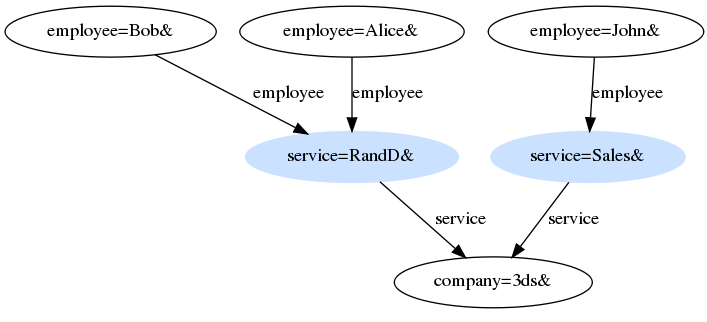
The code for such a transformation may look like the following:
Employee's Transformation Processor
@CVComponentConfigClass(configClass=CVComponentConfigNone.class) public class EmployeeTransformationProcessor implements IJavaAllUpdatesTransformationProcessor { public EmployeeTransformationProcessor(final CVComponentConfigNone config) { } @Override public String getTransformationDocumentType() { return "employee"; } @Override public void process(final IJavaAllUpdatesTransformationHandler handler, final IMutableTransformationDocument document) throws Exception { final String companyName = document.getMeta("company_name"); final String serviceName = document.getMeta("service_name"); if ((companyName != null) && (! companyName.isEmpty()) && (serviceName != null) && (! serviceName.isEmpty())) { final ITransformationDocument serviceDoc = addService(handler, document, serviceName, companyName); document.addArcTo("employee", serviceDoc.getUri()); } } private ITransformationDocument addService(final IJavaAllUpdatesTransformationHandler handler, final IMutableTransformationDocument document, final String serviceName, final String companyName) { final IMutableTransformationDocument newDoc = handler.createDocument("service=" + serviceName + "&", "service"); newDoc.addArcTo("service", "company=" + companyName + "&"); handler.yield(newDoc); // Note that the yield here is required because it is a document created // during the Transformation phase return newDoc; } }
The drawback of this implementation is that it pushes the arcs that link services to the company several times. In the end, since these arcs have the same type, only the relevant ones persist (with no redundancies in the store).
However, it is always better to minimize the number of redundant operations. If we had the required information, we could create the different services that the company has, with unique URIs, and then at the employee level, we would link employees to services.
A possible implementation could be:
... @Override public void process(final IJavaAllUpdatesTransformationHandler handler, final IMutableTransformationDocument document) throws Exception { final String serviceName = document.getMeta("service_name"); if ((companyName != null) && (! companyName.isEmpty()) && (serviceName != null) && (! serviceName.isEmpty())) { document.addArcTo("employee", "service=" + serviceName + "_" + companyName + "&"); } } ...
Despite its imperfection, let us stick to this first implementation from now on. For more information about the method used in this sample, see IMutableTransformationDocument.